- I finally finished my first React app: a Spotify playlist generator! Show /r/reactjs I have been a long time Spotify user and regularly make playlists for different moods, activities or situations.
- Last week, I completed my final project at Flatiron, a React-Redux app with a Rails API. My team decided to also make use of the Spotify API for our app, PlaylistPal, so that visitors could make playlists using the very cool metrics that Spotify stores about each of its songs, including danceability, valence, and amount of vocals.
Let’s address the HomePod Mini Spotify situation. Have Apple confirmed that you can use the popular music app through their new device?
To say that we’ve all been spending more time stuck at home this year would be an understatement.
We are going to get started quickly by running Create React App. You can do this by running the following commands in your terminal. Npx create-react-app react-spotify-player cd react-spotify-player npm start. Open up the project in your favorite text editor and then let’s get our app authenticated to Spotify so we can get that juicy data.
In response to the COVID-19 pandemic, months have been previously spent in full lockdown and many are still avoiding going out unless essential.
Fortunately, a number of things have helped the public adjust, whether that’s taking on new hobbies, watching TV, exercise routines or beyond.
It’s safe to say that many have also finally decided to splash out on some new gadgets for the home.
There are so many products available now designed to make home life a little more luxurious and Apple enthusiasts are currently anticipating the release of the HomePod Mini. Spotify free how many songs on a playlist.
Nevertheless, those who would be lost without Spotify have a pressing question: will the app be available to use on the forthcoming device?
- HUBIE HALLOWEEN: Who was dressed as Billie Eilish?

HomePod Mini Spotify situation explained
Input Mag reports that Apple has not listed Spotify as a third party platform which the HomePod Mini will support.
However, the previous source notes that Spotify can choose to participate, so those wishing to purchase the product will simply have to wait and see whether it’s confirmed.
Inevitably, this uncertainty has become a concern for some, as alternative speaker systems from the likes of Google or Amazon support the popular music service.
In the press release for the HomePod Mini, Spotify isn’t mentioned amongst the third party platforms it will support, so which are?
- HUBIE HALLOWEEN: Adam Sandler’s daughters star!
HomePod Mini: Which music apps are confirmed?
While Spotify hasn’t been confirmed, a number of other services have been.
As highlighted by Input Mag, Apple’s HomePod Mini press release confirms that it “is designed to work with Apple Music, podcasts, radio stations from iHeartRadio, radio.com, and TuneIn, and in the coming months, popular music services including Pandora and Amazon Music.”
So, there are a number of ways that users can access music, podcasts and more through their HomePod Mini.
How much is it?
According to The Verge, the HomePod mini will cost $99 and will be available to preorder from Friday, November 6th 2020.
The product will then begin shipping on Monday, November 16th 2020.
Spotify users will likely be keen to know whether the device will support the streaming service ahead of the preorder date in order to consider purchasing.
As for price comparison, the previous source acknowledges that the original HomePod cost $349 when it launched, making it more expensive than similar products.
On the other hand, they include that it now routinely costs around $199, making the HomePod Mini a no-brainer for those who have been keen to pick up the former model.
In other news, is The Boys cancelled?
Here is the demo and GitHub repository.
Short introduction to React
React is one of the most popular JavaScript libraries for interactive web applications. It enables developers to create reusable UI components and eases to deal with the view layer. It can handle complex updates in applications by manipulating the DOM if necessary. By definition, DOM manipulation is the heart of the modern, interactive web. However, updating DOM is a lot slower than many JavaScript operations. That creates a problem. Especially if we are dealing with huge amounts of DOM manipulation.
Scope of our App
This app generates a simple quiz based on user’s Spotify playlists. Obviously, React relies at the heart of this project. Our fundamental focus was to create a single page React application with below features:
Another excellent option when searching for the best apps like Spotify is GrooveShark. This free music streaming service brings you plenty of playlists to choose from. GrooveShark is internet radio at its. You may also like: 13 Best FM Transmitter Apps for Android and iOS. Back to menu ↑ Deezer Music. Here is the app which allows you to listen to music for free. You can find your own song among 40 million tracks. As long as all your favorite music will be gathered in one place you will feel like. Thankfully, there are lots of great music apps available out there, to ensure you get the best possible experience. Read on for 10 best apps like Spotify that are helping millions of people each day to have access to their favorite the music. The popular website Pandora transitioned to an app. Angela Lang/CNET. Spotify is the pioneer in the music-streaming space arguably the best known. It offers a number of curated music discovery services, including its Discover Weekly playlist,. Get music on spotify free. Stream free albums and hits, find a song, discover music, and download songs and podcasts with the Spotify free streaming and music player app. Free streaming, music search and hits library – Spotify is all that and much more. Play songs, sync music, discover music and free albums with Spotify, your go-to music downloader.
- Connecting to Spotify Web API,
- Accessing provided data,
- Extracting useful information from that data,
- Generating multiple choice questions.
App structure (components and functions)
Each component has its own CSS file. Although it is super useful, we didn’t prefer to use CSS modules, since our app did not require to have unique classnames for every component. By definition, CSS Modules let us use the same CSS class name in different files without worrying about naming clashes. More info here.
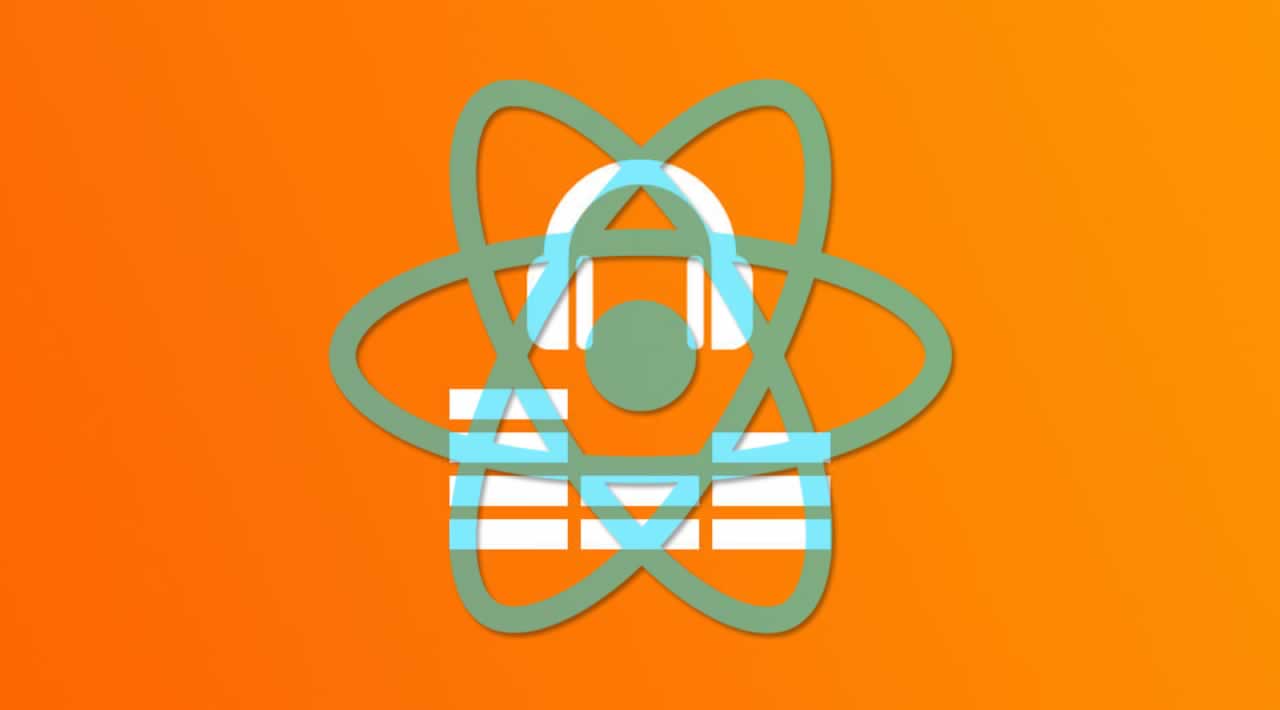
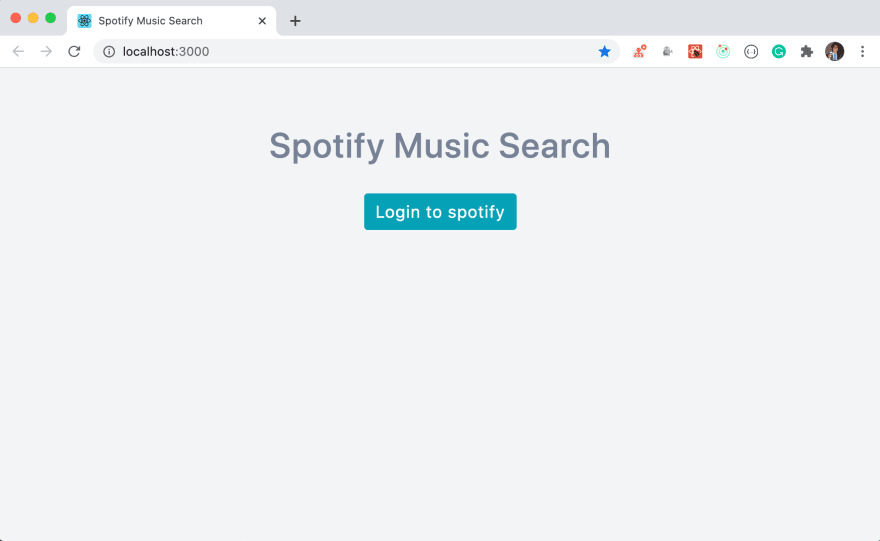
Let’s begin with our main component App.js
. We will analyze the rest in the components breakdown section. This component is the mother of all components. It has one and only resposibility: control the app flow! It is done via receiving props and callback props (react way of passing actions from parent to children).
Here is the flow logic of App.js:
- Render an unchanged header and a footer regardless of the app logic.
- Render
<LoginOrGreeting />
and receive App’s isLogged and token states. - If islogged is true, render
<GetUserPlaylists />
, pass token as props and expect playlist id. Update selectedPlaylist state in the callback. - If playlist id is received, render
<GetPlaylistTracks />
, pass token + selectedPlaylist as props and expect tracks array. Update tracks state in the callback. - If tracks array has a length, render
<CreateQuestions />
, pass tracks array as props. (Last component doesn’t have a callback).
Spotify Web API
Install Spotify App
Spotfiy has a list of libraries for integrating with the Spotify Web API using several programming languages and platforms. We used jmperez/spotify-web-api-js JavaScript library for this project. We first made a node installation and then instantiate the wrapper.
After setting up the environment, we coded our own functions on top of this library. All functions are collected in a single file named spotifyFunctions.js
. We accessed them with import in which component we need:
As it is stated in App structure section, several components share the work load of fetching data & getting useful information out of it. Thus, these components actually become an individual app itself. This is one of the beauties of React, if we ever need to use Spotify user playlists information for some other project, we only need that component and the Web API wrapper (reusable components).
React App With Spotify Subscription
Components breakdown
LoginOrGreeting Component: This component acts as a container for the login screen and greeting. It is the very first component that App.js renders. Initially, isLogged and accessToken states are false and null. Therefore, it first renders
<AccountLogin/>
. Its main responsibility is to decide which component to render and report this to its parent, App.js.Once AccountLogin component renders, we obtain an ugly URL that contains our access_token. In our componentDidMount method, we parse this URL and set it to state (along with isLogged state). At this moment, since we are logged in, our component renders
<Greeting />
and passes accessToken props to it.Note: If we can’t obtain the URL, we won’t have access_token. Therefore, our component would render
<AcountLogin />
again. However, this is not an endless loop! Djay free spotify. Since the generated link in<AcountLogin />
makes the actual login request to Spotify, it has to be clicked by the user.
- AccountLogin Component: This simple presentational component mainly renders the login screen HTML. It is a child of and it’s main responsibility is to make a login request to Spotify on user click. We see this component in action if:
- User logs in for the first time.
- Login is unsuccessfull.
- Spotify session expired. (We currently do not have a condition for that)
- Greeting Component: This is another simple component and a child of
<LoginOrGreeting />
. It renders the welcome message. In addition to that, it displays how many playlists found in users Spotify account. (*Note that, we could split this component further. Currently, it does not only render the HTML, but also fetches name, surname and number of playlists through its lifecycle method).
- GetUserPlaylists Component:
<GetUserPlaylists />
is triggered by isLogged state in App.js (its parent) Its responsibilities are:- Getting user playlists from Spotify.
- Displaying them in a form of HTML select element.
- Making a callback to its parent for the selected playlist id.
- Staying alert for playlist changes made by the user, and repeat above.
- GetPlaylistTracks Component: This component is a pure container component. It has nothing to render so, it is not responsible for any type of UI presentation. It receives accessToken and the selected playlist as props from its parent App.js. It’s resposibilities are:
- Get all tracks of the selected playlist.
- Clean this tracklist data and set state.
- Return this clean array of tracks to the parent,
- Be responsive for playlist changes (if props changes), and repeat above.
- CreateQuestions Component:
<CreateQuestions />
itselft a container component that manages 3 more child presentational components written as stateless functional components. It’s responsibilities are:- Receive myTracks props and pass this tracks array to
prepareQuestions
function. (and set state regarding to returned data) - Manage correct, incorrect state values and pass props to
<Score />
for display. - Pass props to
<QuestionsContainer />
and invoke a callback function when user clicks an answer (onChange event). - Calculate number of remaining questions (the current state), correct / incorrect states.
- Manage
<Result />
and pass the correct state as props. Result component uses props.correct to decide which type of result to display once user finishes the quiz. Moreover, Result component has astart over?
button. Buttons onClick event attribute is attached to a callback function on its parent<CreateQuestions />
, where invokes lifecycle method to request new set of questions for the same playlist.
- Receive myTracks props and pass this tracks array to
See my other React posts: Fetching data from a RESTful API using Fetch API with React and Using React with Bootstrap (react-bootstrap)
React App With Spotify Playlists
Notes, Bugs & References / Reads
- There is too much passing props back and forth. We could think of using React Context API. Context provides a way to pass data through the component tree without having to pass props down manually at every level.
- As we discussed above, App.js is responsible for the main app flow. It handles the flow by props and callbacks. We could use Redux as the state container.
- We could separate our components further. It would allow them to be more reusable and understandable for developer fellows.
- We are aware that there are some bugs in the generated questions. Especially for
prepareQuestionsFunctions.js
:- It does not look for duplicates. i.e.: Imagine a playlist consists of only Metallica songs. In that case, “Who / which group sings….?” question will always have the same answer.
- It does not check for ‘undefined’. It is possible to have undefined answers in questions.
- It does not check number of tracks in the selected playlist. This could cause duplicate questions or same answers. Since we are selecting 10 random indexes from the playlist, any playlist with less than 10 tracks would have duplicate indexes. We may experience this duplication either in questions or answers.
Despite the above-mentioned facts, we didn’t proceed any further with them because it is not in the scope of this project. We mainly focused on receiving data and displaying it using the React environment.
Here are several references and great reads related to this project:
- Passing information to nested components (React.context) Link.
- Updating parent state from a child component Link.
- Query-string Link.
- Async function vs. a function that returns a promise Link.
- Good approach React & Spotify Shuffle Blog Link.
- Spotify referred Web API Wrapper Link.
- Derive states from props Link 1, Link 2.
- Presentational vs. container components Link.
- React Router Link.
Thank you for reading! I would be happy to hear your thoughts and comments.
Here is the demo.
Spotify Free Music App
Here is the GitHub repository.